Appcelerator - ios
Before starting your application instrumentation, ensure that you have created an app and have an appKey
Install
Open tiapp.xml and under modules add the following line
<module version="1.0.0" platform="iphone">ti.pyze<module>
Initialize
- Open tiapp.xml and add the pyze app key as follows
<ios>
<plist>
<dict>
<key>PYZE_APP_KEY<key>
<string><YOUR APP KEY><string>
</dict>
</plist>
</ios>
Warning
Make sure you replacedYOUR_PYZE_APP_KEY
with your own appKey
Import pyze module and get a reference for the module
var pyze = require('ti.pyze');
Custom Events
Custom events allow you to easily track unique user actions within your app.
Events
var pyze = require('ti.pyze');
pyze.postCustomEvent("customEventName")
This allows you to track when a user executed an action, for a simple count of how often that event is occurring.
Events with Attribtues
Attributes are any key-value pair that you can attach to an event. You can have up to 99 attributes with any event. It is highly recommended to follow our Best Practices and heavily rely on attributes to make your instrumentation as minimal and useful as possible. In a typical application, you should have many more attributes than events.
var pyze = require('ti.pyze');
pyze.postCustomEventWithAttributes("customEventName", {"attrrKey1":"attrvalue1", "attrrKey2":"attrvalue2"}
Timed Events
Timing how long a user takes to complete an action is a common usecase for Pyze. The SDk has built-in handling for this, by allowing you to start a timer, and then send a custom event at the completion of that action.
var pyze = require('ti.pyze');
var timerRef = pyze.timerReference
pyze.postCustomEventWithAttributes("customEventName", timerRef, {"attrrKey1":"attrvalue1", "attrrKey2":"attrvalue2"})
User Privacy
Pyze provides APIs to allow end-users to Opt out of Data Collection and also instruct the Pyze system to forget a user’s data.
setUserOptOut
Allows end-users to opt out from data collection. Opt-out can be toggled true or false.
pyze.setUserOptOut(true)
To resume user data collection set value to false
deleteUser
Allows end-users to opt out from data collection and delete the user in the Pyze system. We recommend you confirm this action as once a user is deleted, this cannot be undone.
pyze.deleteUser(true)
Push Notifications
IOS Push Notifications
Apple provides the Apple Push Notification Service (APNS) to allow app publishers to reach out to their users via push notifications.
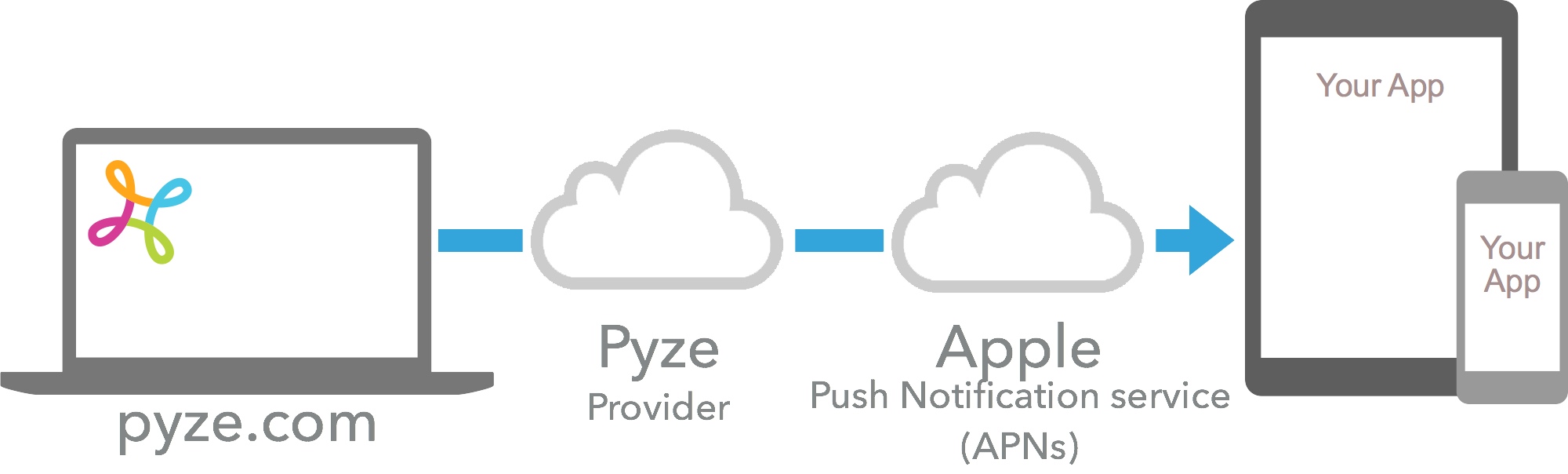
1. Generate P12 Certificate
- Go to
Certificates, Identifiers & Profiles
page of your apple developer account. - Select
Identifiers
and go toYOUR_APP_IDENTIFIER
. - Under
Capabilities
go toPush Notifications
and selectConfigure
- Now under Development/Production SSL Certificate select
Create Certificate
- Upload the
Certificate Signing Request
as mentioned here - Download the certificate generated and add to the
Keychain Access
- Now select the certificate just imported and choose
Export
. - Enter the password and save the
.p12
to the desired location.
2. Configure Pyze as your provider
In order to allow Pyze to send push notifications on your behalf to users of your app, please provide the iOS push certificate to Pyze.
- Login to growth.pyze.com
- Click Settings from the Navigation Pane
- Select the app you want to provide keys for from the drop down list
- Select Push Notifications on the left menu
- Upload a Push notifications certificate in .p12 format, provide p12 password (the password you generated while creating the certificate), and specify the provisioning mode: Development or Production depending on the type of certificate you are using.
- Select daily and weekly quota limits
- Click Save
3. Enabling Push Notifications
Open tiapp.xml
file and add following lines
<ios>
<plist>
<dict>
<key>UIBackgroundModes</key>
<array>
<string>fetch</string>
<string>remote-notification</string>
</array>
</dict>
</plist>
</ios>
Enable your app for Push Notifications
Generate a build for iOS before proceeding.
Enable Remote Notifications
See appcelerator guide for push notification here
Ti.App.iOS.addEventListener('usernotificationsettings', function registerForPush() {
Ti.App.iOS.removeEventListener('usernotificationsettings', registerForPush);
Ti.Network.registerForPushNotifications({
success: deviceTokenSuccess,
error: deviceTokenError,
callback: receivePush
});
});
Register notification types to use
Ti.App.iOS.registerUserNotificationSettings({
types: [
Ti.App.iOS.USER_NOTIFICATION_TYPE_ALERT,
Ti.App.iOS.USER_NOTIFICATION_TYPE_SOUND,
Ti.App.iOS.USER_NOTIFICATION_TYPE_BADGE
]
});
Let Pyze know the APNS device token
Add following code
function deviceTokenSuccess(e) {
deviceToken = e.deviceToken;
var pyze = require('ti.pyze');
pyze.setRemoteNotificationDeviceToken(deviceToken);
}
Let Pyze process Remote Notifications
Add following code
function receivePush(e) {
Ti.API.info('receivePush `'+JSON.stringify(e)+'`:');
// alert('Received push: ' + JSON.stringify(e));
pyze.processReceivedRemoteNotification(e)
}
Interactive Notifications
See appcelerator interactive notifications guide here
Pyze provides a set of predefined push notification categories for showing interactive notifications. Below is the list of categories and respective actions:
Yes or No (opens the app)
Category Id : pyze_bool_foreground
Actions:
1. Title: Yes
Action Id: yes
Foreground: true
Authentication Required: true
2. Title: No
Action Id: no
Foreground: false
Authentication Required: true
Yes or No (dismiss notification)
Category Id : pyze_bool_background
Actions:
1. Title: Yes
Action Id: yes
Foreground: true
Authentication Required: true
2. Title: No
Action Id: no
Foreground: false
Authentication Required: true
Accept or Decline (opens the app)
Category Id : pyze_accept_action_foreground
Actions:
1. Title: Accept
Action Id: accept
Foreground: true
Authentication Required: true
2. Title: Decline
Action Id: decline
Foreground: false
Authentication Required: true
Accept or Decline (dismiss notification)
Category Id : pyze_accept_action_background
Actions:
1. Title: Accept
Action Id: accept
Foreground: false
Authentication Required: true
2. Title: Decline
Action Id: decline
Foreground: false
Authentication Required: true
Shop Now
Category Id : pyze_shop_now
Actions:
1. Title: Shop Now
Action Id: shop_now
Foreground: true
Authentication Required: true
Buy Now
Category Id : pyze_buy_now
Actions:
1. Title: Buy Now
Action Id: buy_now
Foreground: true
Authentication Required: true
Follow
Category Id : pyze_buy_now
Actions:
1. Title: Follow
Action Id: follow
Foreground: false
Authentication Required: true
Opt-In
Category Id : pyze_opt_in
Actions:
1. Title: Opt-In
Action Id: opt_in
Foreground: false
Authentication Required: true
Unfollow
Category Id : pyze_unfollow
Actions:
1. Title: Unfollow
Action Id: unfollow
Foreground: false
Authentication Required: true
Opt-out
Category Id : pyze_opt_out
Actions:
1. Title: Opt-out
Action Id: opt_out
Foreground: false
Authentication Required: true
Remind Me Later
Category Id : pyze_remind_me_later
Actions:
1. Title: Remind Me Later
Action Id: opt_out
Foreground: false
Authentication Required: true
Download
Category Id : pyze_download
Actions:
1. Title: Download
Action Id: download
Foreground: true
Authentication Required: true
Share
Category Id : pyze_share
Actions:
1. Title: Share
Action Id: share
Foreground: true
Authentication Required: true
Download / Share
Category Id : pyze_download_share
Actions:
1. Title: Download
Action Id: download
Foreground: true
Authentication Required: true
2. Title: Share
Action Id: share
Foreground: true
Authentication Required: true
Remind Me Later / Share
Category Id : pyze_remind_share
Actions:
1. Title: Remind Me Later
Action Id: remind
Foreground: false
Authentication Required: true
2. Title: Share
Action Id: share
Foreground: true
Authentication Required: true
Defining a notification category
Ex. Category, Accept or Decline (opens the app) can be defined as below
var acceptAction = Ti.App.iOS.createUserNotificationAction({
identifier: “accept”,
title: “Accept”,
activationMode: Ti.App.iOS.USER_NOTIFICATION_ACTIVATION_MODE_FOREGROUND,
destructive: false,
authenticationRequired: true
});
var rejectAction = Ti.App.iOS.createUserNotificationAction({
identifier: “decline”,
title: “Decline”,
activationMode: Ti.App.iOS.USER_NOTIFICATION_ACTIVATION_MODE_BACKGROUND,
destructive: true,
authenticationRequired: true
});
var acceptDeclineCategory = Ti.App.iOS.createUserNotificationCategory({
identifier: "pyze_accept_action_foreground",
actionsForDefaultContext: [acceptAction, rejectAction],
actionsForMinimalContext: [acceptAction, rejectAction]
});
Like notification types, register defined notification categories by using the Titanium.App.iOS.registerUserNotificationSettings() as below:
Ti.App.iOS.registerUserNotificationSettings({
types: [
Ti.App.iOS.USER_NOTIFICATION_TYPE_ALERT,
Ti.App.iOS.USER_NOTIFICATION_TYPE_SOUND,
Ti.App.iOS.USER_NOTIFICATION_TYPE_BADGE
],
categories: [acceptDeclineCategory]
});
Monitor Interactive Notifications
Let pyze process the notification received and perform selected push action:
Ti.App.iOS.addEventListener('remotenotificationaction', function(e) {
Ti.API.info('remotenotificationaction `'+JSON.stringify(e)+'`:');
pyze.handlePushNotificationAction(e.identifier, e);
});
}
Rich Notifications
Open the .xcodeproj and follow the instruction in below link in-order to integrate rich push notifications
In-App Notifications
In-app notifications allow app businesses to reach out to app users when they use your app.
In-App notifications are built-in to the pyze sdk and have no additional dependecies.businesses to reach out to users from manually from Dynamic Funnels and Intelligence Explorer, and automatically based on workflows and campaigns from Growth Automation.
In-App notifications can be sent from Campaigns
Call the following method, wherever you want to check for new messages and pop-up a modal with the notification:
void showInAppNotificationUIForDisplayMessagesWithCustomAttributes(messageType, buttonTextcolor, buttonBackgroundColor, backgroundColor, messageCounterTextColor, callback);
Usage
var pyze = require('ti.pyze');
pyze.showInAppNotificationUIForDisplayMessagesWithCustomAttributes(0, "#ffffff", "#ffffff", "#ffffff", "#ffffff", function(params){
var status = params[0]
Ti.API.info('InAppStatus `'+params[0]+'`:');
});
Debugging and Logging
Please refer to the ios documentation for platform-specific information
API Reference
Full API reference available here