Appian
Before starting your application instrumentation, ensure that you have created an app and have an appKey
Install
Install the Pyze Ananytics Component from Appian AppMarket and ensure it is enabled in your list of plugins.
Initialize
In your application create a common Expression Rule for Pyze Analytics Component (ex: PDPyze) to make this component easily accesible from your applications pages.
Paste the following code inside Expression Rule Component
/*Get pyze app key from https://growth.pyze.com */
{
pyze(
pyzeAppKey: "YOUR_PYZE_APP_KEY",
methodName: ri!methodName,
eventName: ri!eventName,
attributes: ri!attributes,
userId: ri!userId,
pyzeProfileAttributes: ri!pyzeProfileAttributes,
customProfileAttributes: ri!customProfileAttributes,
/*randomString: (rand()-0.5)/500*/
)
}
Warning
Make sure you replacedYOUR_PYZE_APP_KEY
with your own appKey
Create the input variables methodName, eventName, attributes, userId, pyzeProfileAttributes, and customProfileAttributes as shown below.
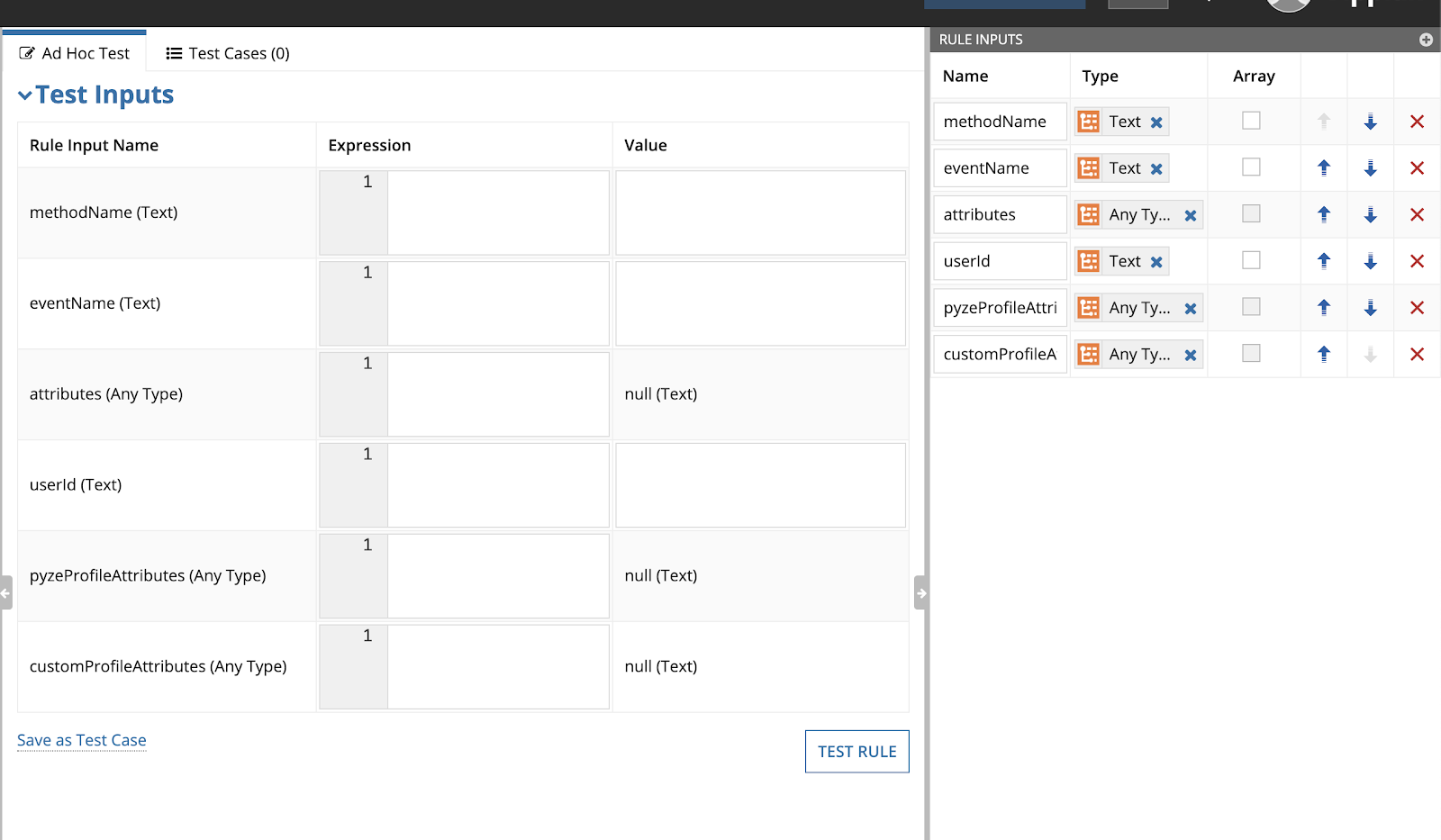
Calling initialize
is required on each page that you want to track, add the local variable to your interfaces, and and make sure method name is set to initialize
.
This will ensure that when page is loaded initialize method is invoked, and the pyze SDK will begin collecting data.
local!methodName : "initialize",
local!eventName : "",
local!attributes : {},
local!userId:"",
local!pyzeProfileAttributes:{},
local!customProfileAttributes:{},
Add the expression rule to each of interface of your application.
rule!PDPyze(
methodName: local!methodName,
eventName: local!eventName,
attributes: local!attributes,
userId: local!userId,
pyzeProfileAttributes: local!pyzeProfileAttributes,
customProfileAttributes: local!customProfileAttributes
)
Optionally, you can omit varibles if you aren’t using them on that page. Ex: if we aren’t calling profile API, above snippet would look like this.
/*
* Local variables
*/
local!methodName : "initialize",
local!eventName : "",
local!attributes : {},
...
/*
* Component with fewer variables.
*/
rule!PDPyze(
methodName: local!methodName,
eventName: local!eventName,
attributes: local!attributes
)
Custom Events
Events allow you to easily track unique user actions within your app.
Invoke the Pyze API by updating local variables inside saveIntos
.
Event
// Custom Event without Attributes
saveInto: {
a!save(local!methodName,"postCustomEvent"),
a!save(local!eventName, "YOUR_EVENT_NAME")
}
This allows you to track when a user executed an action, for a simple count of how often that event is occurring.
Event with Attributes
Attributes are any key-value pair that you can attach to an event. You can have up to 99 attributes with any event. It is highly recommended to follow our Best Practices and heavily rely on attributes to make your instrumentation as minimal and useful as possible. In a typical application, you should have many more attributes than events.
// Custom Event with Attributes
// Create an object with key value pairs of custom attributes and post with postCustomEventWithAttributes
saveInto: {
a!save(local!methodName,"postCustomEventWithAttributes"),
a!save(local!eventName, "YOUR_EVENT_NAME"),
a!save(local!attributes, {“attr1”:”value1”, “attr2”:123})
}
Timed Events
Timing how long a user takes to complete an action is a common usecase for Pyze. The SDk has built-in handling for this, by allowing you to start a timer, and then send a custom event at the completion of that action.
startTimerForEvent
- Start the timer with a namepostTimedEvent
- End the timer by referencing the same name. The time in milliseconds will automatically be attached to this event. You can also optionally pass attributes as an additional paramater as show in the example below
//Start timer for event
saveInto: {
a!save(local!methodName,"startTimerForEvent"),
a!save(local!eventName, "YOUR_EVENT_NAME")
}
//Post timed event.
//Note : The event name attribute for the `startTimerForEvent` and `postTimedEvent` should match.
saveInto: {
a!save(local!methodName,"postTimedEvent"),
a!save(local!eventName, "YOUR_EVENT_NAME")
}
//Post timed event API when you want to send additional attributes
saveInto: {
a!save(local!methodName,"postTimedEventWithAttributes"),
a!save(local!eventName, "YOUR_EVENT_NAME"),
a!save(local!attributes, {“attr1”:”value1”, “attr2”:123})
}
Dimensions
Dimensions are attributes that can be attached to all events within a session. This is useful to have a standard set of attributes that change over time, and is simply a helper to accomplish this goal.
Dimensions are any key-value pairs that you attach to the initialize
call. Modify the initialize
call to include these keypairs or json object
dimensions: {"dim1":"val1"}
Profile
Profiles are a powerful way to add data about your users to Pyze. Use profiles to add data that is not specific to a point in time (events) and are attached to a specific user.
When a user logs in to your app, call setUserProfile
to identify them to Pyze. Any events that happen after they are identified, will be attributed to this user. You should only call this method once upon the initial login of a session.
You can optionally include profile attributes.
Pyze built-in profile attributes get special handling in the pyze UI, and we will attempt to discern the data type for custom attributes based on the data recieved. There is no difference between the built-in attributes and custom ones outside of formatting applied.
//Create Pyze Profile Attributes object
saveInto: {
a!save(local!methodName,"setUserProfile"),
a!save(local!userId, "USER_UNIQUE_IDENTIFIER"),
a!save(local!pyzeProfileAttributes, {“first_name”:”John”),
a!save(local!customProfileAttributes, {“city”:”XYZ”)
}
Pyze profile attributes
Pyze User Profile Field Name | Data Type/Description |
---|---|
background | (string) User background, biography or historical data |
country | (string) Country codes must be sent in the ISO-3166-1 alpha-2 standard. |
current_location | (object) Format: {“longitude”: -33.991894, “latitude”: 25.243732} |
date_of_first_use | (date at which the user first used the app) String in ISO 8601 format or in yyyy-MM-dd’T’HH:mm:ss.SSSZ format. |
date_of_last_use | (date at which the user last used the app) String in ISO 8601 format or in yyyy-MM-dd’T’HH:mm:ss.SSSZ format. |
date_of_birth | (date of birth) String in format “YYYY-MM-DD”, example: 1984-06-01. |
email_id | (string) Email Id |
email_subscribe | (string) Acceptable values are “opt_in” (explicit approval to receive email messages), “opt_out” (explicit denial to email messages), and “subscribed” (neither opted in nor out). |
email_hard_bounced | Automatically updated when a hard bounce occurs (true or false) |
email_spam_reported | Automatically updated when a user marks your email as spam, via the ISP (true or false) |
facebook_id | facebook ID |
first_name | (string) User’s First name |
gender | (string) “M”, “F”, “O” (other), “N” (not applicable), “P” (prefer not to say) or “U” (unknown). |
home_city | (string) User’s Home City |
image_url | (string) URL of image to be associated with the user |
language | (string) Require language to be sent in the ISO-639-1 standard. |
last_name | (string) User’s Last Name |
marked_email_as_spam_at | (string) Date at which the user’s email was marked as spam. Must be in ISO 8601 format or in yyyy-MM-dd’T’HH:mm:ss.SSSZ format. |
phone | (string) Phone number |
push_subscribe | (string) Available values are “opted_in” (explicitly registered to receive push messages), “unsubscribed” (explicitly opted out of push messages), and “subscribed” (neither opted in nor out). |
push_tokens | Array of objects with app_id and token string. You may optionally provide a device_id for the device this token is associated with, e.g., [{“app_id”: App Identifier, “token”: “abcd”, “device_id”: “optional_field_value”}]. If a device_id is not provided, one will be randomly generated. |
time_zone | (string) Time Zone’s must be sent as per IANA Time Zone Database (e.g., “America/New_York” or “Eastern Time (US & Canada)”). Only valid values will be respected. |
twitter_id | Twitter ID |
Updating an existing user Profile
To update user profile attributes which are already set, use the following api.
Note : Do not call this api before calling setUserProfile.
saveInto: {
a!save(local!methodName,"updateUserProfile"),
a!save(local!pyzeProfileAttributes, {“first_name”:”John”),
a!save(local!customProfileAttributes, {“city”:”XYZ”)
}
Logging out a user
Call this api when a user logs out. Event sent after this call will have no identity attached, and will be attributed to an anonymous user.
saveInto: {
a!save(local!methodName,"resetUserProfile")
}
User Privacy
Pyze provides APIs to allow end-users to Opt out of Data Collection and also instruct the Pyze system to forget a user’s data.
setUserOptOut
Allows end-users to opt out from data collection. Opt-out can be toggled true or false.
saveInto: {
a!save(local!methodName,"setUserOptOutToTrue")
}
To resume user data collection set value to false
deleteUser
Allows end-users to opt out from data collection and delete the user in the Pyze system. We recommend you confirm this action as once a user is deleted, this cannot be undone.
saveInto: {
a!save(local!methodName,"deleteUser")
}